How to Get Google Analytics
Session ID and Session Number
A little bit of reverse engineering
goes a long way.
Published on
-/- lines long
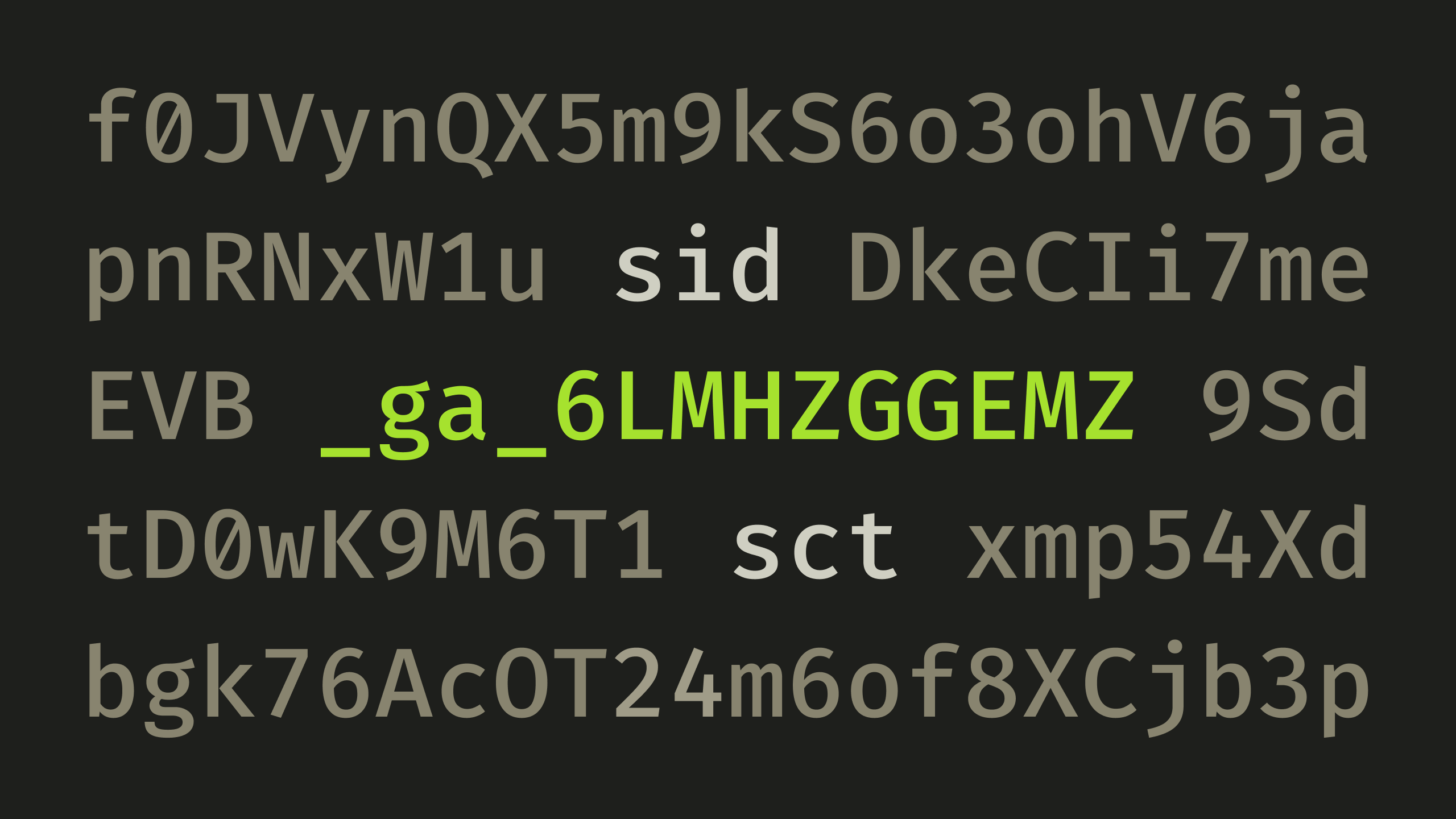
If you're building your own custom analytics integration using Segment(opens in new tab), for example, you might need to obtain the GA4 session ID and session number. Otherwise, your events won't be appearing in the GA4 dashboard.
As Google states in their GA4 docs about sessions(opens in new tab):
When a session starts, Google automatically collects a
session_start
event and generates a session ID (ga_session_id
) and session number (ga_session_number
) via the session_start(opens in new tab) event.
The problem is that those two values don't seem to be programmatically accessible. However, if we look at the source code of the gtag.js script on my site(opens in new tab), for example, we can see suspicious key names:
yb:"session_id",
pe:"session_number",
If we then search for those yb
and pe
keys, we can see that they're getting mapped to sid
and sct
:
qA[T.g.yb] = "sid";
qA[T.g.pe] = "sct";
…and where do those names appear? In the AJAX ping whenever a new event is being sent:
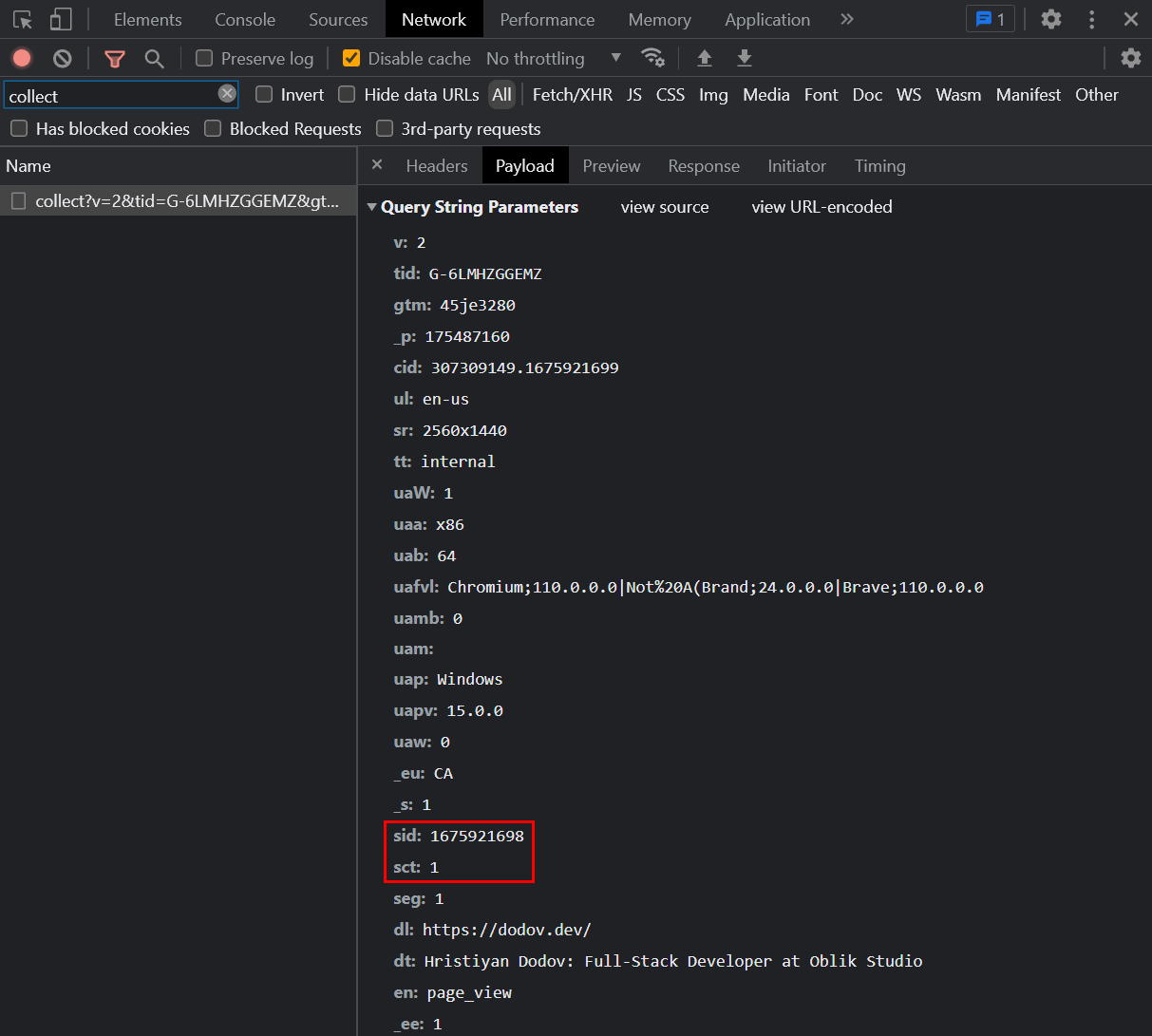
But GA surely has to persist those values on the browser between page loads. That's the point of sessions, after all. And yes, if we inspect one of the _ga
cookies, we can find the values there:
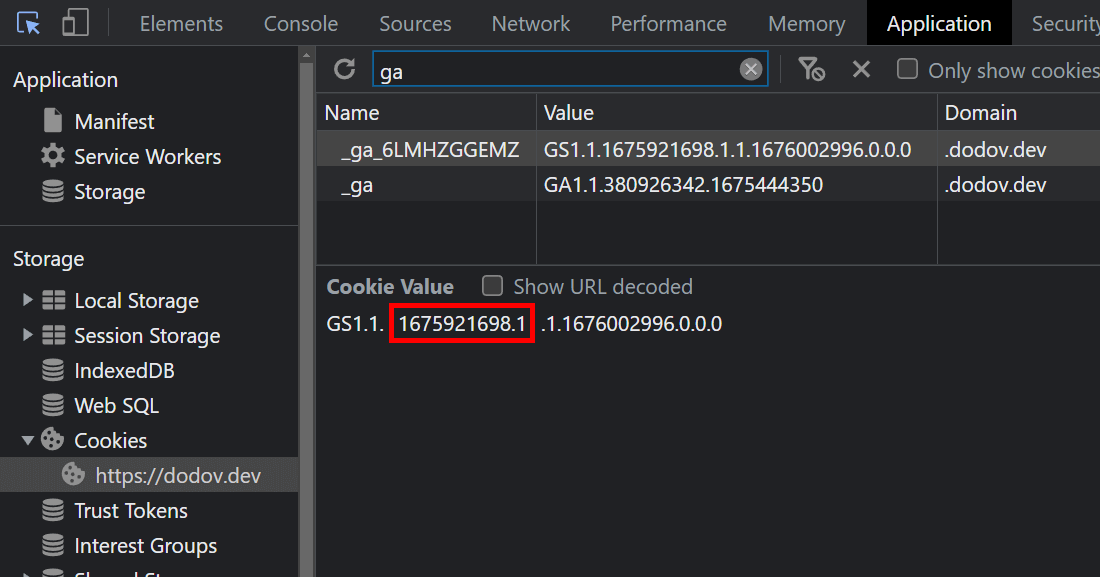
There are many digits "1" in that cookie, but I'm sure that the one specifically after the session ID (marked in red above) is used for the session number. When the session number increases, that digit in the cookie changes accordingly.
Now, if we want to obtain those session values, we just have to parse that _ga
cookie:
function getSessionData(callback) {
// Use your own ID here ↓
const pattern = /_ga_6LMHZGGEMZ=GS\d\.\d\.(.+?)(?:;|$)/;
const match = document.cookie.match(pattern);
const parts = match?.[1].split(".");
if (!parts) {
// Cookie not yet available; wait a bit and try again.
window.setTimeout(() => getSessionData(callback), 200);
return;
}
callback({
ga_session_id: parts.shift(),
ga_session_number: parts.shift(),
});
}
Note that the function is polling the cookie, because if your script runs before Google Analytics has had the chance to load, it won't be able to retrieve the values. When the values are available, the callback function you pass will be invoked with an object argument that looks like this:
{
ga_session_id: "1675921698",
ga_session_number: "1",
}
You can then pass the session data to your underlying systems.